How to make a python __init__ file
• • ☕️ 2 min readHow to make a python init file
If you’ve used Python programming language for long enough, you undoubtedly must’ve came across a file named init.py In this quick guide we will give a practical example of the usage of such a file.
Practical example:
lets say you have a couple of functions that you use in your code, and you wanted to move these functions to another file and import them in your code. let’s say that you put this code in a file called main_file.py
def helper_function1(a,b):
return a + b
def helper_function2(a,b):
return a - b
x = helper_function1(1,2)
y = helper_function2(1,2)
print(x)
print(y)
and your project folder now contains only this file like the following image
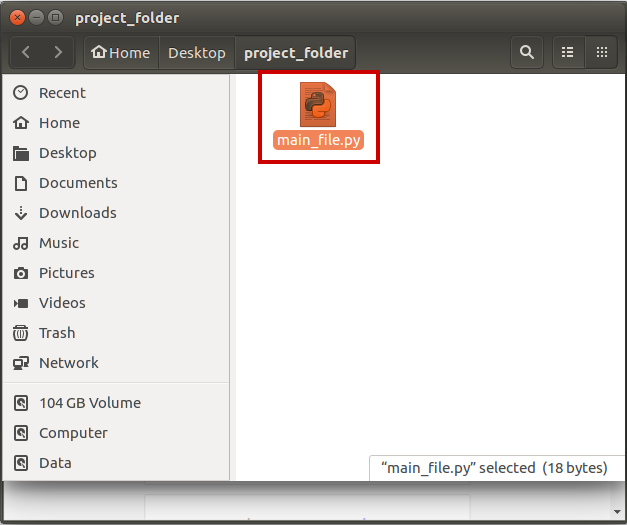
You want to change the code by putting helper_function1 and helper_function2 in a separate file and import them like the following:
from another_file import helper_function1,helper_function1
x = helper_function1(1,2)
y = helper_function2(1,2)
print(x)
print(y)
and our files structure would become:
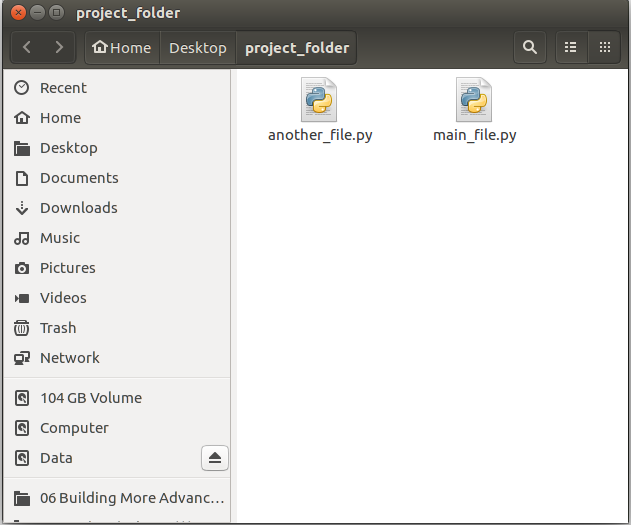
and you can import the needed functions like so:
from another_file import helper_function1,helper_function1
x = helper_function1(1,2)
y = helper_function2(1,2)
A probable scenario:
Imagine you wanted to organize your files by making a directory that you put some helper modules in like the following images:
a directory for the helper modules:
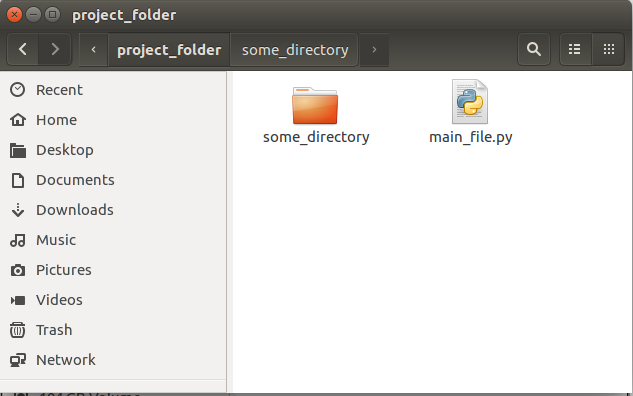
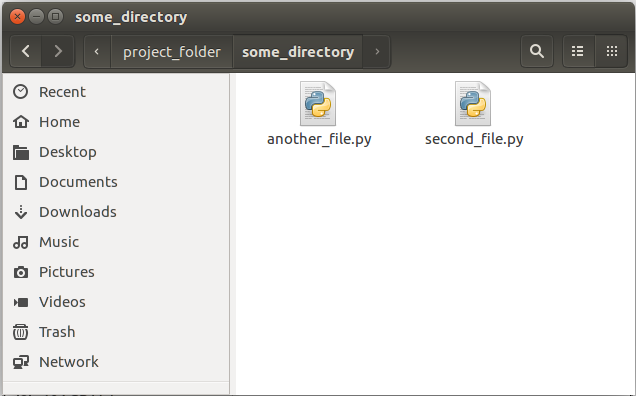
in that case you need to create an empty init.py file inside the helper modules directory like the following:
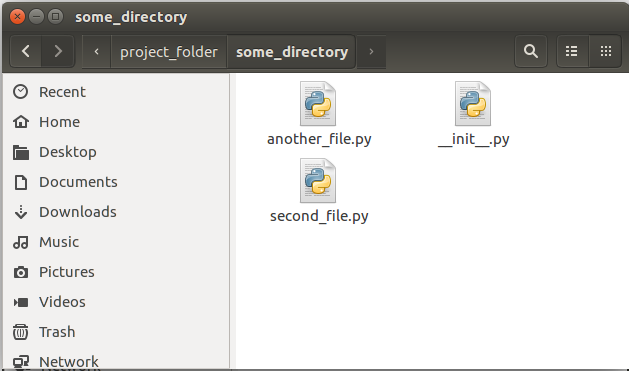
notice that the init is completely empty, all you need is to create it
after creating the empty init file inside the directory you want to import from, you can import the items you need like the following:
from some_directory import another_file, second_file
OR:
from some_directory.another_file import *
from some_directory.second_file import *